Laravel env
Install & Config JWT
install tymon/jwt-auth
1
|
composer require tymon/jwt-auth 1.0.0-rc.3
|
Generate the JWT_SECRET
secret key to .env
, it used to sign the tokens
config/app.php
add providers
and aliases
:
1
2
3
4
5
6
7
8
9
10
|
<?php
'providers' => [
Tymon\JWTAuth\Providers\LaravelServiceProvider::class,
]
'aliases' => [
'JWTAuth' => 'Tymon\JWTAuth\Facades\JWTAuth',
'JWTFactory' => 'Tymon\JWTAuth\Facades\JWTFactory',
]
|
關於aliases : 引述這篇說的一段話
如果你不使用这两个 Facade,你可以使用辅助函数 auth()
auth() 是一个辅助函数,返回一个guard,暂时可以看成 Auth Facade。
对于它有很多有必要说的,可以看我单独写的一篇文章——Laravel 辅助函数 auth 与 JWT 扩展详解
Configure Auth guard in config/auth.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
<?php
'defaults' => [
'guard' => 'api',
'passwords' => 'users',
],
...
'guards' => [
'api' => [
'driver' => 'jwt',
'provider' => 'users',
],
],
|
modify app/Http/Middleware/Kernel.php
1
2
3
4
5
6
|
<?php
protected $routeMiddleware = [
'jwt.auth' => \Tymon\JWTAuth\Http\Middleware\Authenticate::class,
'jwt.refresh' => \Tymon\JWTAuth\Http\Middleware\RefreshToken::class,
];
|
publish the package config file, and you will find jwt.php
in your config/
1
|
php artisan vendor:publish --provider="Tymon\JWTAuth\Providers\LaravelServiceProvider"
|
Update your User model
modify app/User.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
<?php
namespace App;
use Tymon\JWTAuth\Contracts\JWTSubject;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable implements JWTSubject
{
use Notifiable;
// Rest omitted for brevity
/**
* Get the identifier that will be stored in the subject claim of the JWT.
*
* @return mixed
*/
public function getJWTIdentifier()
{
return $this->getKey();
}
/**
* Return a key value array, containing any custom claims to be added to the JWT.
*
* @return array
*/
public function getJWTCustomClaims()
{
return [];
}
}
|
PS : class User
need to implements JWTSubject
Create User data
使用tinker
工具快速建立測試使用者
1
2
|
>>> namespace App;
>>> User::create(['name' => 'Test','email' => 'test@example.com','password' => bcrypt('12345')]);
|
update routes
modify routes/api.php
1
2
3
4
5
6
7
8
9
10
11
12
|
<?php
Route::post('/login', 'AuthController@login');
Route::middleware('jwt.auth')->group(
function () {
Route::get('/logout', 'AuthController@logout');
Route::get('/me', 'AuthController@me');
}
);
Route::get('/token/refresh', 'AuthController@refresh');
|
Add own Facade class
app/Libs/Facade/Common.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
<?php
namespace App\Libs\Facade;
use Symfony\Component\HttpKernel\Exception\HttpException;
class Common extends \Illuminate\Support\Facades\Facade
{
public static function jsonResponse(array $payload = null, $statusCode = 200, $header = [])
{
$payload = $payload ?: [];
return response()->json($payload, $statusCode, $header, JSON_UNESCAPED_UNICODE);
}
public static function jsonErrorResponse($message = null, $code = null, $statusCode = null, $headers = [])
{
$payload = [
"error" => [
"message" => $message,
"code" => $code,
]
];
return response()->json($payload, $statusCode, $headers, JSON_UNESCAPED_UNICODE);
}
}
|
update Controllers
app/Http/Controllers
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
|
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use Symfony\Component\HttpKernel\Exception\BadRequestHttpException;
use Symfony\Component\HttpKernel\Exception\UnauthorizedHttpException;
use App\Exceptions\InternalServerHttpException;
use Validator;
use JWTAuth;
use App\Libs\Facade\Common;
class AuthController extends Controller
{
protected function respondWithToken($token)
{
return Common::jsonResponse(
[
'access_token' => $token,
'token_type' => 'Bearer',
'expires_in' => JWTAuth::factory()->getTTL() * 60
], 200
);
}
public function login(Request $request)
{
$credentials = $request->only('email', 'password');
$rules = [
'email' => 'required|email',
'password' => 'required',
];
$validator = Validator::make($credentials, $rules);
if ($validator->fails()) {
throw new BadRequestHttpException($validator->messages(), null, 101, []);
}
if (! $token = JWTAuth::attempt($credentials)) {
throw new UnauthorizedHttpException('jwt', 'Login error, email or password failed!', null, 102, []);
}
return $this->respondWithToken($token);
}
public function logout(Request $request)
{
$token = $request->header('Authorization');
JWTAuth::invalidate($token);
return Common::jsonResponse(
[
'message' => 'Successfully logged out',
], 200
);
}
public function refresh(Request $request)
{
return $this->respondWithToken(JWTAuth::parseToken()->refresh());
}
public function me(Request $request)
{
return response()->json(
[
"user" => $request->user(),
"auth" => JWTAuth::payload()
]
);
}
}
|
update Exceptions
app/Exceptions/Handler.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
<?php
public function render($request, Exception $e)
{
$message = $e->getMessage();
$headers = [];
$code = 9999;
$statusCode = 500;
if ($e instanceof HttpException) {
$headers = $e->getHeaders();
$code = $e->getCode();
$statusCode = $e->getStatusCode();
}
// Override exception message
$exp = get_class($e);
switch ($exp) {
case 'Symfony\Component\HttpKernel\Exception\NotFoundHttpException':
$message = "page not found";
break;
default:
# code...
break;
}
return Common::jsonErrorResponse($message, $code, $statusCode, $headers);
// Origin code...
//return parent::render($request, $e);
}
|
特別說明一下Exception
這個部分 :
在實作jwt功能之前,研究很多別人的寫法
總覺得拋 api 回傳結果寫的不是很好
如果你也有Google一些jwt的文章
你會發現很多人都會直接response->json(…, 200)回傳api結果
我這邊針對這個問題做了一些個人調整(不一定是最好的,歡迎批評指教~)
render
: 判斷Exception是否為HttpException
型態,若是
則可以call getStatusCode()等方法,若否
則StatusCode 一律回傳 500
Result
/api/login : 使用者登入,得到jwt token
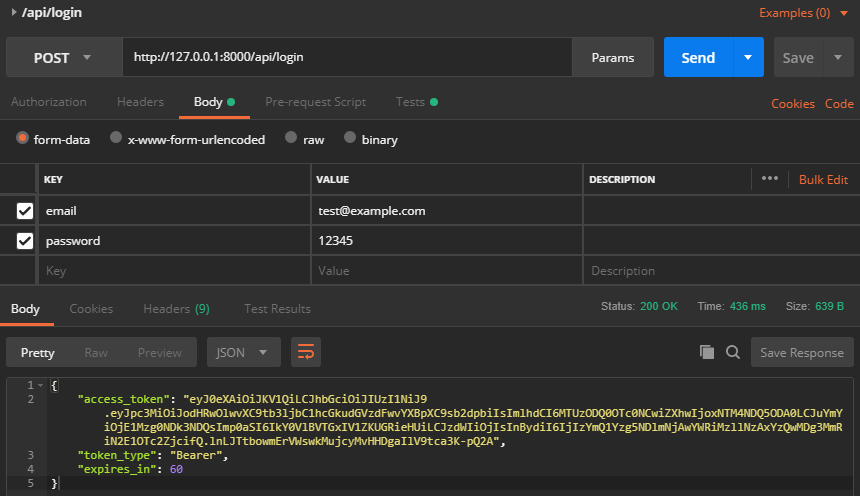
/api/me : 顯示使用者資訊,注意headers需要使用Authorization
: Bearer xxxxxxxxxxxxxxxxxxxxx
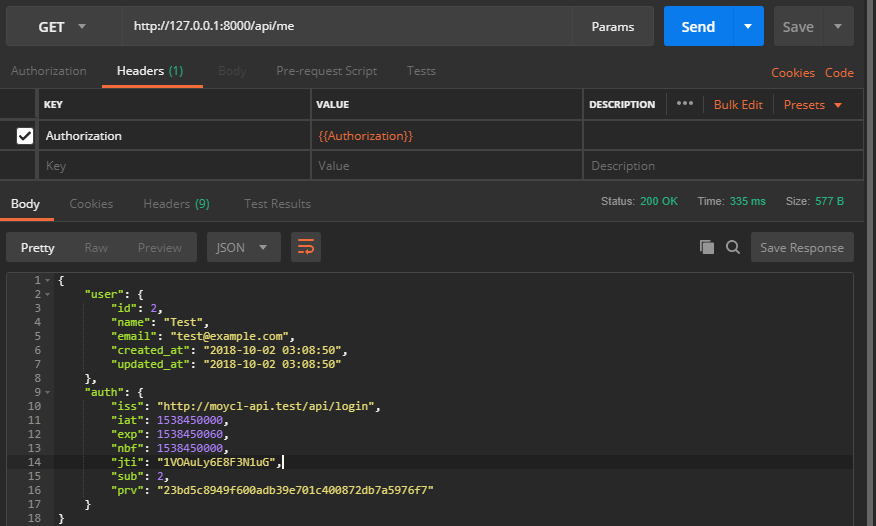
可以到config/jwt.php
, 將ttl
設定為 1
mins,比較容易測試Token has expired
的狀況
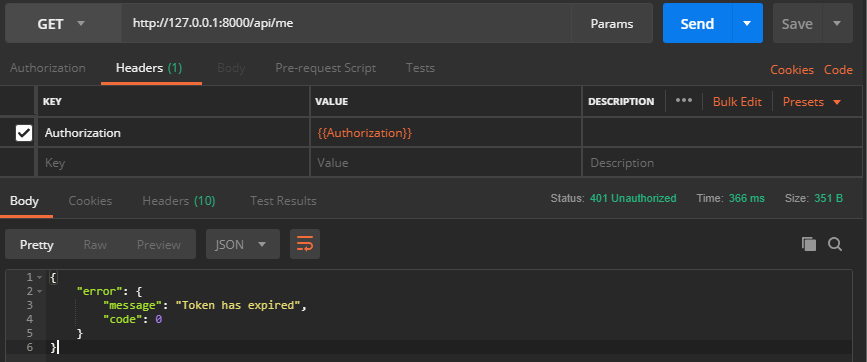
/api/logout : 將jwt token註銷,如果再使用此token,則會出現401 : The token has been blacklisted
的錯誤
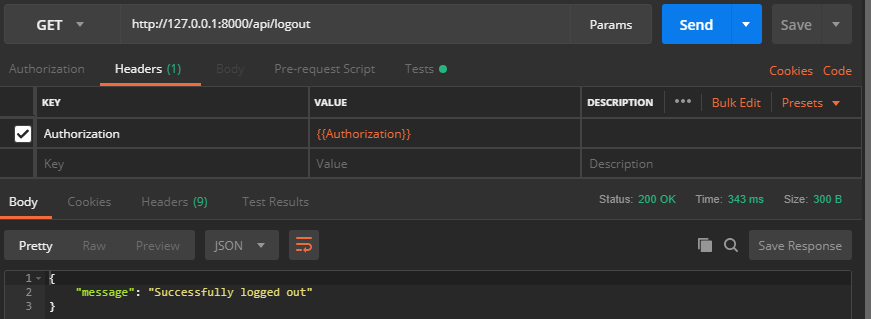
Conclusion
最後補充一下Exception的小東西
Exception裡面有一個code
這個參數
在實作大型api專案時
因為api很多,相對的api各類錯誤類型也爆炸多
透過throw new Exception() 時
我們可以把自定義的api錯誤代碼放入code
裡
統一由處理Exception的function顯示api錯誤代碼 → 個人覺得還不錯用
如果有更好整理code的方法 歡迎在下方留言 ↓↓↓
Reference